Overview
Creating a connector allows you to integrate APIs outside of Demyst with Demyst's platform, Data APIs, and providers.
Doing so is a two step process. First, you define your external API using a custom JSON definition language (details below) and provide inputs for testing. Demyst will make a request to that API, and use that to generate a more detailed JSON definition, which we'll call the schema.
Second, you review the generated schema as well as the initial response Demyst received from the API. If both look good, you can confirm the creation of your new connector. You're free to further alter the schema at this time, but generally shouldn't have to.
Getting Started
Step 1: Create connector
To start, use the menu on the left-hand side to choose "Create Connector". Here you will supply a unique name for the connector and the JSON definition of the external API.
You can explore the details of that JSON definition here.
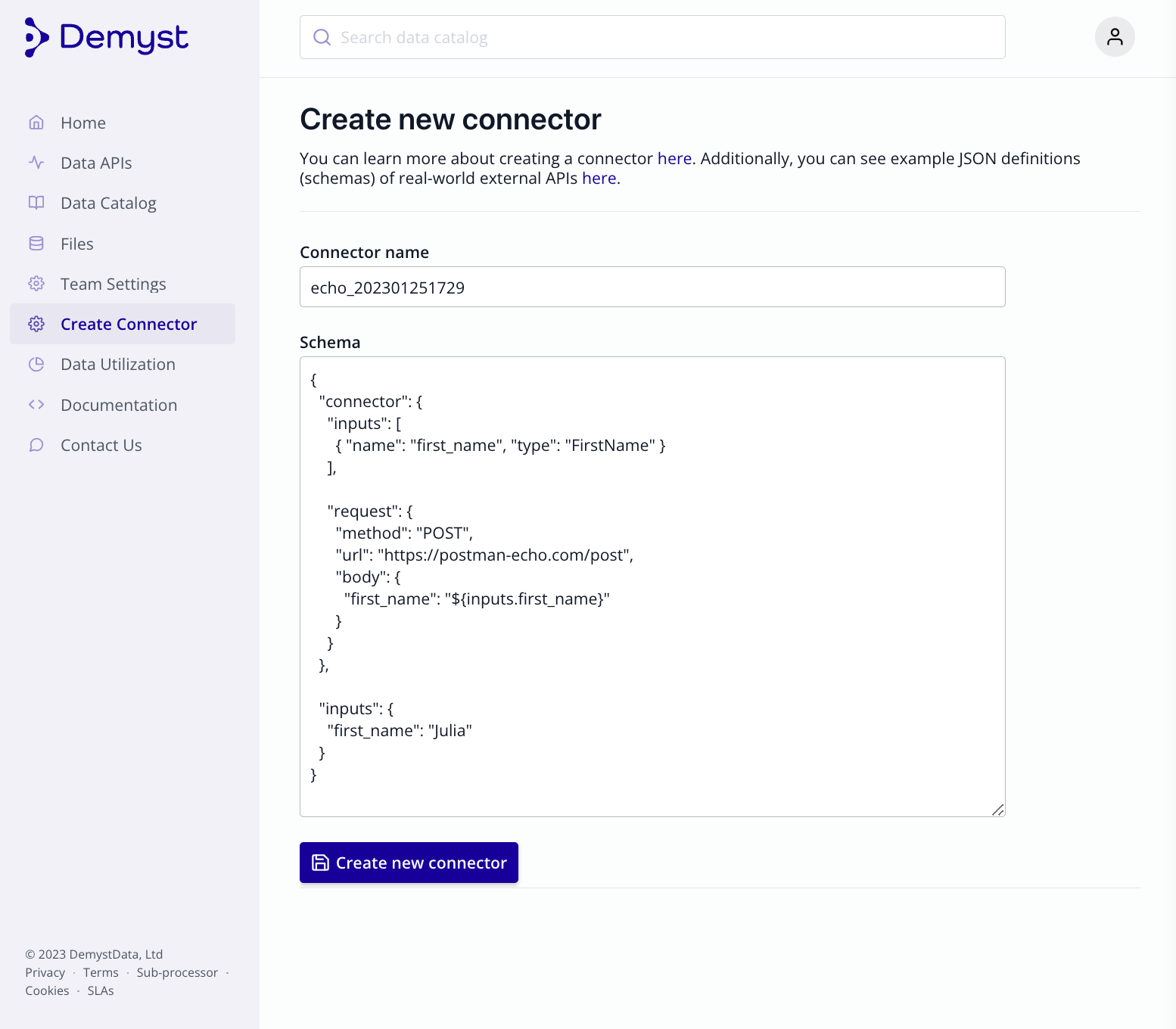
Step 2: Verify and Confirm Connector
After Demyst makes an intial request to the API you described in Step 1, you review the schema we generated and the initial response we received. If they look good, confirm the connector creation.
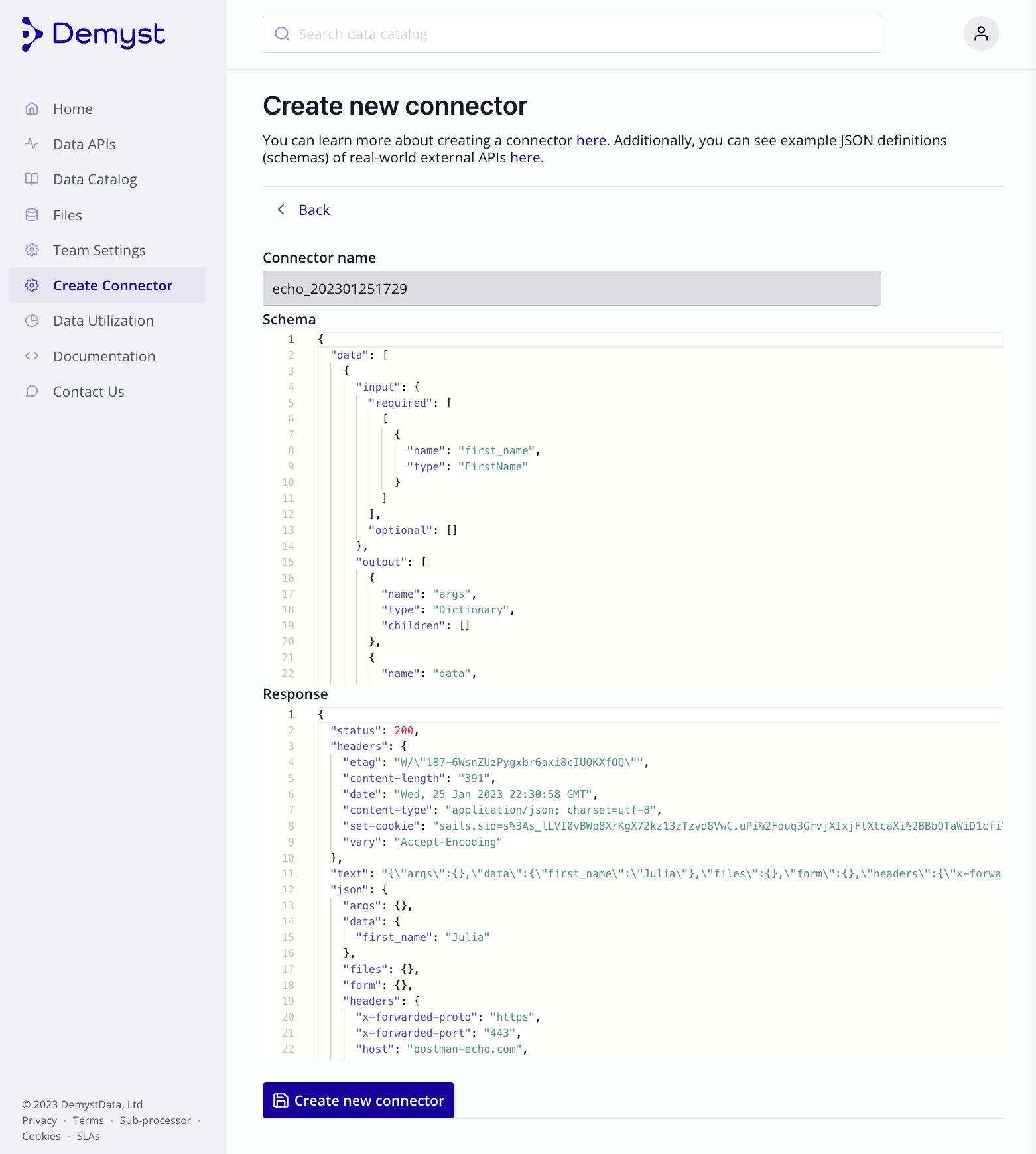
JSON Definition
connector.inputs
Required list of objects specifying inputs that can be used in string interpolation. name and type are required. type needs to be a known demyst type.
connector.credentials
Optional list of credentials that can be used in string interpolation.
request.method
One of GET | POST.
request.url
The URL of the external API. Credentials and inputs can be interpolated in this string, for example: "https://example.com?api_token=${credentials.api_token}&first_name=${inputs.first_name}".
request.headers
An object describing headers to include in the request. Credentials and inputs can be interpolated in the header values. This is probably most useful setting an Authorization header, for example: { "Authorization": "Bearer ${credentials.token}" }.
request.body
An object describing the JSON body to use for POST requests. Credentials and inputs can be interpolated in any string values.
inputs
Inputs to use when making an initial request to the external API to validate request logic and generate a more detailed JSON definition.
Real-World API Examples
JsonWHOIS
{
"connector": {
"inputs": [
{ "name": "domain", "type": "Domain" }
],
"request": {
"method": "GET",
"url": "https://jsonwhois.com/api/v1/whois?domain=${inputs.domain}",
"headers": {
"Authorization": "Token token=hard_coded_token"
}
}
},
"inputs": {
"domain": "demystdata.com"
}
}
JsonWHOIS (with interpolated credential)
{
"connector": {
"inputs": [
{ "name": "domain", "type": "Domain" }
],
"credentials": ["api_token"],
"request": {
"method": "GET",
"url": "https://jsonwhois.com/api/v1/whois?domain=${inputs.domain}",
"headers": {
"Authorization": "Token token=${credentials.api_token}"
}
}
},
"inputs": {
"domain": "demystdata.com"
},
"credentials": {
"api_token": "interpolated_token"
}
}
Relativity 6
{
"connector": {
"inputs": [
{ "name": "address", "type": "Street" },
{ "name": "state", "type": "State" },
{ "name": "country", "type": "Country" }
],
"request": {
"method": "POST",
"url": "https://api.relativity6.guru/naics/naics/search",
"headers": {
},
"body": {
"token": "secret",
"name": "${inputs.name}",
"address": "${inputs.address}",
"state": "${inputs.state}",
"advancedSearch": true,
"prediction": true,
"existenceCheck": true,
"keywords": true,
"inputWarning": true,
"explainConfidenceScore": true
}
}
},
"inputs": {
"address": "TX",
"state": "TX"
}
}
MaxMind Insights
{
"connector": {
"inputs": [
{ "name": "ip_address", "type": "IP4" },
{ "name": "email_address", "type": "EmailAddress" }
],
"request": {
"method": "POST",
"url": "https://minfraud.maxmind.com/minfraud/v2.0/insights",
"headers": {
"Authorization": "Basic hard-coded-in-this-example"
},
"body": {
"device": {
"ip_address": "${inputs.ip_address}"
},
"email": {
"address": "${inputs.email_address}"
}
}
}
},
"inputs": {
"ip_address": "17.178.96.59",
"email_address": "help@demystdata.com"
}
}
Telesign
{
"connector": {
"inputs": [
{ "name": "phone", "type": "String" }
],
"request": {
"method": "POST",
"url": "https://rest-ww.telesign.com/v1/phoneid/${inputs.phone}",
"headers": {
"Authorization": "Basic secret"
},
"body": {
"addons": { "device_info": {} }
}
}
},
"inputs": {
"phone": "18884521505"
}
}
Postman's Echo API
{
"connector": {
"inputs": [
{ "name": "first_name", "type": "FirstName" }
],
"request": {
"method": "POST",
"url": "https://postman-echo.com/post",
"body": {
"first_name": "${inputs.first_name}"
}
}
},
"inputs": {
"first_name": "Julia"
}
}